12 Cuda Memcpy Async Tricks For Faster Transfers
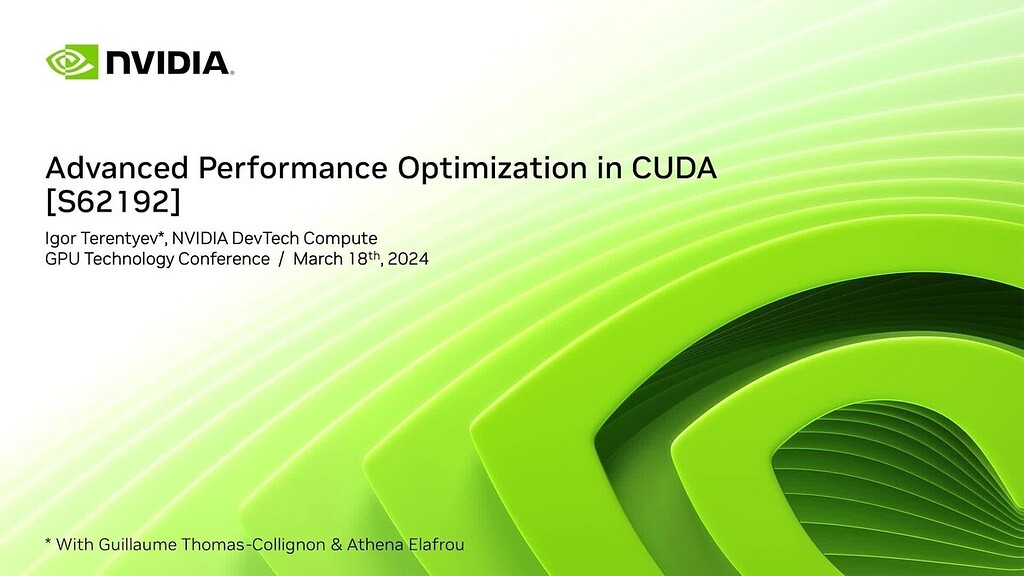
The art of optimizing data transfers in CUDA, a crucial aspect of harnessing the full potential of NVIDIA’s GPUs for general-purpose computing. At the heart of efficient data movement between the host and device memories lies the cudaMemcpyAsync
function, a powerful tool for overlapping data transfers with computations, thereby minimizing idle time and maximizing throughput. However, mastering the use of cudaMemcpyAsync
requires a deep understanding of its intricacies and the environment in which it operates. Here are 12 tricks to help you squeeze the most out of cudaMemcpyAsync
for faster data transfers.
1. Understanding CUDA Streams
Before diving into cudaMemcpyAsync
, it’s essential to grasp the concept of CUDA streams. Streams are sequences of commands (kernel launches, memory transfers) that are executed in order on the GPU. Understanding how streams work and using them effectively is crucial for asynchronous data transfers. Each stream can execute independently, allowing for the overlap of data transfers and computations.
2. Synchronization with cudaDeviceSynchronize
When using cudaMemcpyAsync
, you might need to ensure that all previously launched commands on a stream have completed. cudaDeviceSynchronize
is a blocking call that waits for all commands in all streams to complete. However, for finer-grained control, consider using cudaStreamSynchronize
for synchronizing within a specific stream.
3. Profiling Tools for Optimization
NVIDIA provides powerful profiling tools like Nsight Systems and Nsight Graphics. These tools can help you understand the execution timeline of your application, identifying bottlenecks and areas where cudaMemcpyAsync
can be more effectively utilized to overlap data transfers with kernel executions.
4. Pinning Host Memory
For efficient asynchronous data transfer, consider pinning the host memory using cudaHostAlloc
. Pinned memory is page-locked, meaning it cannot be swapped out, which reduces the time required for data transfer and can significantly improve performance when using cudaMemcpyAsync
.
5. Choosing the Right Memory Type
CUDA provides various memory types (e.g., page-locked host memory, device memory, managed memory) each with its characteristics. Understanding these types and selecting the most appropriate one for your specific use case can significantly impact the performance of cudaMemcpyAsync
.
6. Memory Coalescing for Efficient Transfers
While primarily a consideration for kernel development, ensuring that data is laid out in memory to facilitate coalesced access can also influence the efficiency of data transfers. Properly structured data can reduce the number of transactions required for transfer, potentially improving the performance of asynchronous operations.
7. Batching Transfers for Reduced Overhead
Instead of making numerous small transfers, batching data can reduce the overhead associated with each transfer operation. This approach can lead to more efficient use of the PCIe bus and the GPU’s DMA engines, potentially increasing throughput.
8. Using cudaMemcpy2DAsync
for 2D Transfers
When dealing with 2D arrays, cudaMemcpy2DAsync
can provide better performance than cudaMemcpyAsync
by allowing more efficient transfer of pitch-linear memory. This is particularly useful in scenarios where 2D data structures are common, such as in image processing.
9. Handling Errors with cudaGetLastError
While not directly a performance optimization, monitoring and handling errors gracefully is crucial for the reliability of applications using cudaMemcpyAsync
. cudaGetLastError
can be used to check for errors after each CUDA call.
10. Awareness of PCIe Bandwidth Limitations
The bandwidth of the PCIe interface can become a bottleneck for data transfers between the host and GPU. Understanding these limitations and planning data transfers accordingly can help in avoiding unnecessary bottlenecks when using cudaMemcpyAsync
.
11. Employing Peer-to-Peer Transfers
In systems with multiple GPUs, using peer-to-peer memory transfers can significantly reduce the latency associated with data movement between GPUs, compared to transferring data through the host. This can be particularly beneficial in clustered environments or when working with distributed datasets.
12. Continuous Monitoring and Adjustment
The performance landscape of GPU-accelerated applications can change with new hardware generations, driver updates, and shifts in workload characteristics. Regularly profiling and adjusting your application to leverage the latest best practices for cudaMemcpyAsync
and other CUDA functions can help in maintaining optimal performance.
FAQ Section
What is the primary benefit of using `cudaMemcpyAsync` over `cudaMemcpy`?
+The primary benefit of using `cudaMemcpyAsync` is its ability to perform memory transfers asynchronously, allowing for the overlap of data transfers with kernel executions, which can significantly improve the overall performance of GPU-accelerated applications.
How can I ensure that `cudaMemcpyAsync` operations are properly synchronized?
+To ensure proper synchronization of `cudaMemcpyAsync` operations, use `cudaStreamSynchronize` for stream-specific synchronization or `cudaDeviceSynchronize` for device-wide synchronization. Additionally, consider using CUDA events for more fine-grained control over synchronization.
What role does pinned memory play in enhancing the performance of `cudaMemcpyAsync`?
+Pinned memory, allocated using `cudaHostAlloc`, is page-locked and cannot be swapped out by the operating system. This characteristic reduces the time required for data transfer and can significantly improve the performance of `cudaMemcpyAsync` by minimizing the overhead associated with page faults.
In conclusion, mastering the use of cudaMemcpyAsync
is a multifaceted endeavor that involves not only understanding the nuances of asynchronous data transfers but also being well-versed in broader CUDA programming principles and practices. By applying these tricks and continuously refining your approach based on the specific requirements of your application and the evolving landscape of GPU computing, you can unlock significant performance improvements and create more efficient, scalable applications.